Application Development - C/C++ Example
Introductionβ
This article describes the main steps for developing C/C++ applications for Torizon OS devices using the Torizon IDE extension. It presents a walkthrough of the development process. You will learn to go from a project template to a working application. To help you test and understand the basics, we will also guide you in setting up and running a sample that uses the GPIO interface.
This article covers the following topics:
- Install the C/C++ development environment.
- Details about the sample.
- Install C/C++ libraries.
- Give containers access to devices.
- Deploy and debug projects.
- Next steps.
This article complies with the Typographic Conventions for Torizon Documentation.
Prerequisitesβ
- Get a Toradex Hardware with Torizon OS installed.
- Set up the Torizon IDE extension Environment.
- Connect a Torizon OS Target Device.
Create a New Projectβ
Create a new project from the C/C++ Application template. The Torizon IDE extension automatically generates the project folder and the essential files.
Install the C/C++ Development Environmentβ
When working with C/C++ projects, we need to install some C/C++ development tools.
During the first load of a new project, the notification Do you want to check for dependencies on your system?
will appear. Click Yes
.
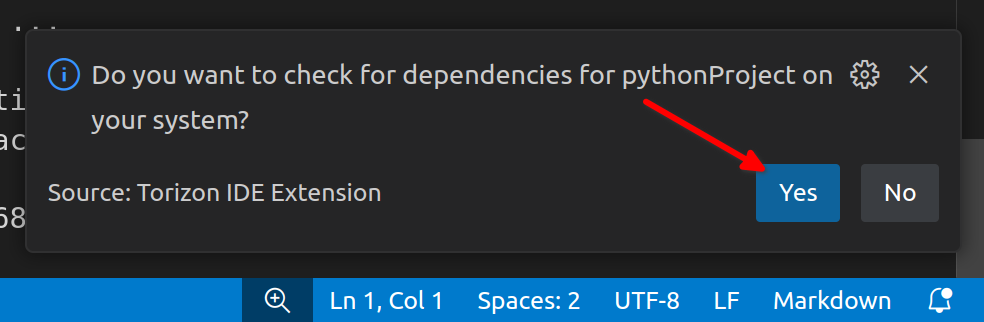
The extension will then trigger the check-deps
task, which checks the necessary local packages (on the development host, such as SDKs) to build the project. For more information, see Check Host Machine Dependencies Required by the Project.
If you don't see the notification, you can manually trigger the check-deps
task. For more information, see Workspace - Tasks.
Confirm the installation and your sudo
password will be requested.
The Sampleβ
This sample consists of a C application that can have two behaviors:
- Continuously toggle a GPIO pin.
- If no pins are defined in the code, the application prints all GPIO pins available in the terminal.
The entire project is available on GitHub. For more information about this and other samples provided by Toradex, see Samples.
Hardware Setupβ
To test the GPIO pin, you can utilize either a multimeter or an LED to verify the logic level of the connector. When using an LED, ensure you match the voltage and current of both the LED and the GPIO pin. If necessary, consider using a driver circuit to power the LED correctly.
The Verdin Development Board already provides LEDs with driver circuits for quick evaluation:
Thus, we can directly connect the GPIO pin to the carrier board's LED interface, as follows:
Note that the GPIO_3
pin of the Verdin iMX8M Plus, accessible via the SODIMM_210
pin on the Verdin Development Board, was used. If you have different hardware and are unsure where to begin, refer to the How to Use GPIO on Torizon OS article.
Source Codeβ
The code uses the gpiod
C library to interact with GPIO pins, abstracting hardware complexities. With this setup, we can control GPIO pins with high-level code.
To run this sample, copy the source code below and paste it into the main.c
file of your project.
main.c source code
C / C++ Packages Installationβ
While developing your projects, you may need to add C/C++ libraries that are not part of the default C/C++ library. The IDE Extension uses Makefile to automate the build and cross-compilation process.
To include external libraries:
- Add the library package to the
torizonPackages.json
file. - Link the library to your application: Add it to the LDFLAGS variable of the Makefile. For example:
CC := gcc
CCFLAGS := -Iincludes/
DBGFLAGS := -g
- LDFLAGS :=
+ LDFLAGS := -l<library-1> -l<library-2> -l<library-3>
CCOBJFLAGS := $(CCFLAGS) -c
ARCH :=
To get the gpiod
library working, proceed as follows:
Add the
libgpiod-dev
package to the container image, modifying thetorizonPackages.json
file:torizonPackages.json{
"deps": [
+ "libgpiod2"
],
"devDeps": [
+ "libgpiod-dev"
]
}Note we added
libgpiod2
instead oflibgpiod-dev
todeps
because we do not need static libraries and headers in the runtime container.Link the library program, modifying the Makefile:
CC := gcc
CCFLAGS := -Iincludes/
DBGFLAGS := -g
- LDFLAGS :=
+ LDFLAGS := -lgpiod
CCOBJFLAGS := $(CCFLAGS) -c
ARCH :=
For detailed information, refer to the Add Dependencies and Tools to Existing Projects article.
Peripheral Accessβ
Projects that utilize peripherals require additional configuration. You need to specify the devices that the application container will access. On Linux-based OSes like Torizon OS, devices are available in user space through the /dev
directory. For more information, see Peripheral Access Overview.
There are two methods to make devices accessible within the container:
- Add flags to the Docker Run command.
- Create a Docker Compose file.
The Torizon IDE extension manages the creation and running of containers. Additionally, it automatically creates the docker-compose.yml
file in the project directory, so you only need to add the necessary parameters.
As an example, the following docker-compose.yml
allows the container to access GPIO banks. The highlighted lines show the required configuration:
Docker Compose file for the GPIO Sample
Note that there are two services:
gpioc-debug
: Contains configurations of the debug container. Here we added thevolumes
anddevice_cgroup_rules
properties to allow the container to access all GPIO banks, easing the debugging process.gpioc
: Builds the release container image. Here, we only give the container access to the GPIO bank (/dev/gpiochip0
) used in our source code. It is a security best practice to provide more granular permissions to release containers. For more information about this service, see Build, Test and Push Applications for Production.
Command-Line Argumentsβ
Depending on the development phase of your project, whether you are debugging or have already built a container image, the process of sending arguments to applications varies. For detailed information, refer to the Pass Arguments to Containerized Applications article.
Deploy and Debugβ
After configuring your project, you can deploy and debug it. Since this is common to all languages supported by the extension, please refer to the Deploy and Debug article.
Next Stepsβ
Check out the following articles for an walkthrough on other programming languages.
Toradex also provides a lot of other code samples in different languages and different use cases that can be a good starting point for your applications. For more information see, Samples.
With the Torizon IDE extension, you can also:
- Create a release container image (optimized image with no debugging configurations and dependencies).
- Push images to container registries.
- Push applications to Torizon Cloud.
For more information, see Build, Test and Push Applications for Production.